Over a year ago I had an… incident… with my Dropbox. All of my folders were still present, including nested directories, but in several of those locations all of the files had been surreptitiously deleted. Once I realized this I went straight to the web tool and attempted recovery of the files. I have no idea when they were removed but it turns out that the plan I was paying for originally covered unlimited backups (or something to that effect) but after a few rounds of price changes I was left with only the data that was still in there. Thankfully a large portion of my work relies on git so I didn't lose a lot of progress but I did lose a few things that were important.
One of these things was a java library that I was working on. It was really more of a toolbox than a library; meant to support the stuff I had been doing voraciously for quite a while in Processing. I had been working out of my sketches directory under "libraries" and had initialized a git repository but in my haste had never pushed it anywhere. I'd been dutifully making commits to this thing and (I'd imagine) had expected that it was included in the main repository but, sadly, it wasn't.
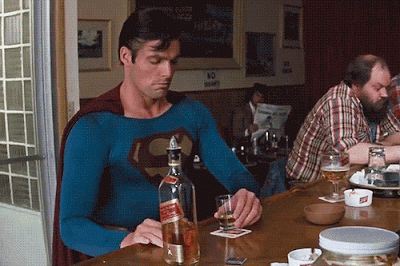
This pretty effectively put the kibosh on my enthusiasm for "creative coding" for the following, well, forever. None of my existing sketches that did interesting things would work because the library that they depended on for data layering or custom "compareTo" were dust.
This month, I decided that I was going to head back into the desert of procedural art, even if that didn't mean sharing a sketch output every day like I used to. As soon as I started I cracked some knuckles and built a really simple pixel sorter; "Okay, yeah, I remember how to do this stuff." But as soon as I went a level deeper I remembered the old library and got sad. I remembered that setting it up last time to be "dog-foodable" was a total pain in the butt and the old "idonwannu" started kicking in. Yesterday I decided that if I was going to do this then dammit it was worth doing right, with sharp tools, and confirmed version control this time.
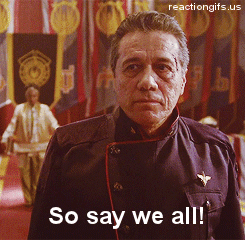
So now, instead of working on a new sketch, I'm writing this blog post. I'm hoping that it makes the process of getting up-and-running easier for one person out there thinking about creative coding. I know that I would have liked a guide for "this is what works for me" when getting set up.
Step 1: Install Processing
Head over to http://processing.org and hit the Downloads section. I'm on a Mac so everything in this guide will be macOS specific. The Java build stuff should apply to any platform however.
Please consider donating a few dollars to this amazing project. It helps a ton of people learn how to write code.
Once you've got Processing.app in your /Applications folder you should be able to run it and tell Gatekeeper that you know what you're doing opening an executable from the internet. This will make a sketch by default and if you save it your processing "sketchbook" folder will be created automatically.
Head up to the "Sketch" menu item and select "Import Library -> Add Library" - select something harmless like "Box2d" so that your libraries folder will be created as well.
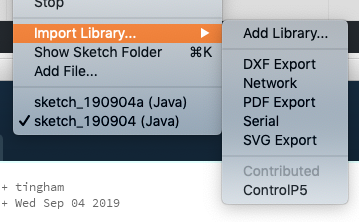
If you want to play around with a sketch, because this is your first time, or because it's addictively fun, there are some great tutorials on the processing.org website that you can follow along with.
As a last step in the Processing app you'll need to install the processing-java
binary. It's under the Tools menu.
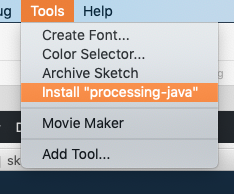
Step 2: Install Homebrew
Because I want to write my own Java library that I can reference from inside of my sketches; I needed to get an up-to-date Apache/Ant installation. If you're reading this you probably already have homebrew installed on your machine but if you don't now is a great time to grab that. It's possible that the default ant
will work just fine but I had already updated to a newer version through brew by running (in a Terminal):
$brew install ant
Once that's done you can:
cd ~/Documents/Processing/libraries
mkdir mylibrary
cd mylibrary
mkdir src
mkdir lib
mkdir build
mkdir library
touch build.xml
touch README.md
git init
After that open up build.xml in your text editor of choice and paste in the following:
<project name="mylibrary" default="dist" basedir=".">
<description>
Java library for processing.org
</description>
<!-- set global properties for this build -->
<property name="src" location="src"/>
<property name="build" location="build"/>
<property name="dist" location="library"/>
<path id="project.class.path">
<pathelement location="lib/core.jar" />
</path>
<target name="init">
<!-- Create the time stamp -->
<tstamp/>
<!-- Create the build directory structure used by compile -->
<mkdir dir="${build}"/>
</target>
<target name="compile" depends="init"
description="compile the source">
<!-- Compile the Java code from ${src} into ${build} -->
<javac srcdir="${src}" destdir="${build}">
<classpath refid="project.class.path" />
</javac>
</target>
<target name="dist" depends="compile"
description="generate the distribution">
<!-- Create the distribution directory -->
<mkdir dir="${dist}/lib"/>
<jar jarfile="${dist}/mylibrary.jar" basedir="${build}"/>
</target>
<target name="clean"
description="clean up">
<!-- Delete the ${build} and ${dist} directory trees -->
<delete dir="${build}"/>
<delete dir="${dist}"/>
</target>
</project>
You can replace "mylibrary" with whatever you want to name your lib. My source src
directory includes a com
and com/urbanfort
because I'm pedantic sometimes and apparently I like changing directories a lot.
The next step is a little weird and I didn't find any information about how to do it differently on any website but basically, you want to find your Processing.app bundle in Finder, then right click on it and "Show Package Contents"
Inside of the bundle you'll find "Java/core.jar" and you will want to copy that file, and then paste it into ~/Documents/Processing/libraries/mylibrary/lib
so that you can reference the processing package from inside your Java classes. After that you can head back to Terminal and try:
ant dist
If that fails please let me know because I've forgotten a step and need to improve the quality of this how-to.
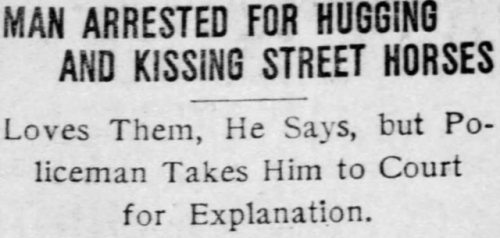
Step 3: Optional Tooling
I've written a script that I use to auto-generate new sketches for myself. I don't really care for the Processing editor itself.
msketch.js is the script I've written to create a sketch folder, pde file and subdirectories. There is a sample command for your vimrc that binds F5 to running the currently open sketch. I'm sure that writing something for bbedit would be similarly simple.